In this example, you will know how to implement api authentication in Laravel 5.4 using JWT with example.
This is very important to implement authentication in web application.
JWT (JSON Web Token) is usually used to send information that can be trusted and verified by means of a digital signature.
Now question is when should you use JSON Web Tokens ?
This is very common scenario for all the web application where you need to set restriction over request, you allow user to access services, resources and interaction with the database with the help of security token and JSON Web Tokens are a best way to transfer information between parties in secure way.
JWT allow these all feature to apply api authentication and normally used in HTTP Authorization headers.
Using JWT is a good way to apply security on your RESTful API services that can be used to enter into your database.
Install the JWT handler packageIn this step, I will install the tymon/jwt-auth
package for api authentication.
Run following command to install package :
composer require tymon/jwt-authUpdate the config/app.php for JWT package
In this step, I will update the config/app.php to add service provider and their aliase.
'providers' => [ .... 'Tymon\JWTAuth\Providers\JWTAuthServiceProvider', ], 'aliases' => [ .... 'JWTAuth' => 'Tymon\JWTAuth\Facades\JWTAuth' ],
Now publish the JWT configuration file, once you have successfully published then you will see a new file created in following path config/jwt.php
.
To publish the configuration file in Laravel you need to run following line of code :
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\JWTAuthServiceProvider"
Now for token encryption, I need to generate a secret key by running following line of code :
php artisan jwt:generateAdd route
In this step, I will define routes for register a new user, login with user credentials and get the authenticated user details by using token.
routes/api.php
- Route::post('auth/register', 'UserController@register');
- Route::post('auth/login', 'UserController@login');
- Route::group(['middleware' => 'jwt.auth'], function () {
- Route::get('user', 'UserController@getAuthUser');
- });
As you can see in above routes, I used middleware so If successfully authenticated then you will get user details from the database.
The main aspect of this tutorial will be on how I can generate JWTs on the back-end (Laravel) side and obtain them on the front-end and then pass the generated token with each request to the API.
Ok, Now I will create middleware to check if the token is valid or not and also You can handle the exception if the token is expired.
php artisan make:middleware VerifyJWTToken
Using this middleware, you can filter the request and validate the JWT token.
Now open your VerifyJWTToken
middleware and put below line of code.
- <?php
- namespace App\Http\Middleware;
- use Closure;
- use JWTAuth;
- use Tymon\JWTAuth\Exceptions\JWTException;
- use Symfony\Component\HttpKernel\Exception\UnauthorizedHttpException;
- class VerifyJWTToken
- {
- /**
- * Handle an incoming request.
- *
- * @param \Illuminate\Http\Request $request
- * @param \Closure $next
- * @return mixed
- */
- public function handle($request, Closure $next)
- {
- try{
- $user = JWTAuth::toUser($request->input('token'));
- }catch (JWTException $e) {
- if($e instanceof \Tymon\JWTAuth\Exceptions\TokenExpiredException) {
- return response()->json(['token_expired'], $e->getStatusCode());
- }else if ($e instanceof \Tymon\JWTAuth\Exceptions\TokenInvalidException) {
- return response()->json(['token_invalid'], $e->getStatusCode());
- }else{
- return response()->json(['error'=>'Token is required']);
- }
- }
- return $next($request);
- }
- }
The try block in handle method check if requested token is verified by JWTAuth or not if it is not verified then exception will be handled in catch block with their status.
Now register this middleware in your kernal to run during every HTTP request to your application.
app/Http/Kernel.phpprotected $routeMiddleware = [ ... 'jwt.auth' => \App\Http\Middleware\VerifyJWTToken::class, ];Create UserController
In this step, I will create a controller "UserController.php" to register a user and login with the registered user.
app/Http/Controllers/UserController.php
- <?php
- namespace App\Http\Controllers;
- use Illuminate\Http\Request;
- use App\Http\Requests;
- use App\Http\Controllers\Controller;
- use JWTAuth;
- use App\User;
- use JWTAuthException;
- class UserController extends Controller
- {
- private $user;
- public function __construct(User $user){
- $this->user = $user;
- }
- public function register(Request $request){
- $user = $this->user->create([
- 'name' => $request->get('name'),
- 'email' => $request->get('email'),
- 'password' => bcrypt($request->get('password'))
- ]);
- return response()->json(['status'=>true,'message'=>'User created successfully','data'=>$user]);
- }
- public function login(Request $request){
- $credentials = $request->only('email', 'password');
- $token = null;
- try {
- if (!$token = JWTAuth::attempt($credentials)) {
- return response()->json(['invalid_email_or_password'], 422);
- }
- } catch (JWTAuthException $e) {
- return response()->json(['failed_to_create_token'], 500);
- }
- return response()->json(compact('token'));
- }
- public function getAuthUser(Request $request){
- $user = JWTAuth::toUser($request->token);
- return response()->json(['result' => $user]);
- }
- }
Now let's check the API response with Postman.
1 : I will first register a user so that i can login with the help of user credentials.
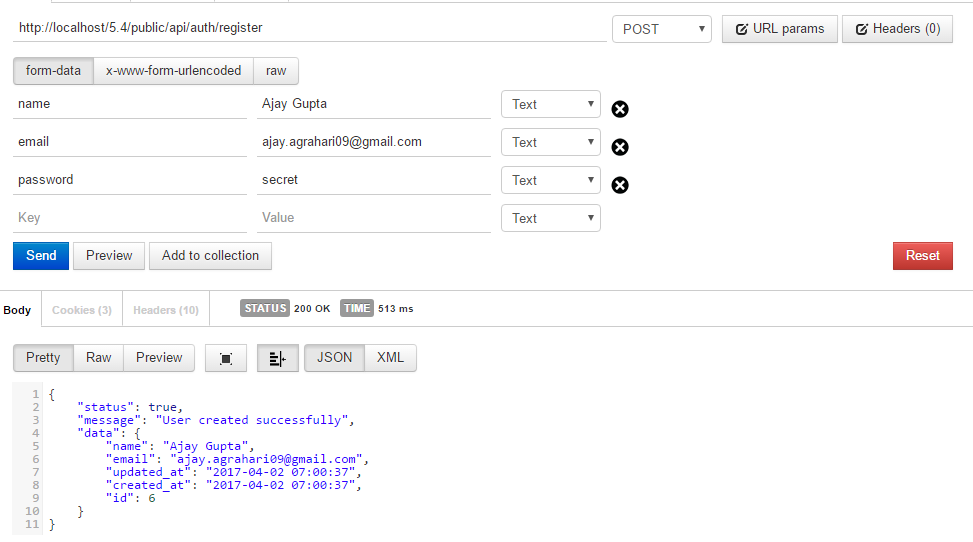
2 : Now I will login with the credentials to get a token :
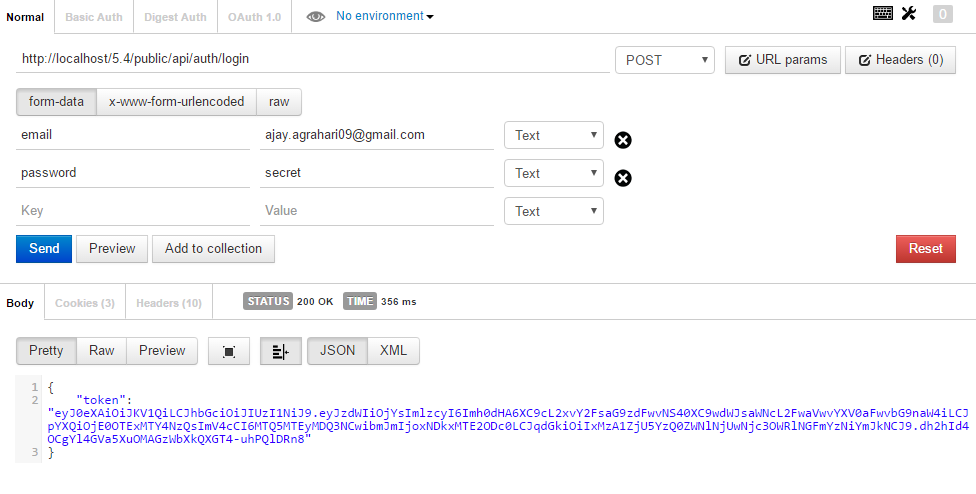
3 : Now I will hit the api to get user details :
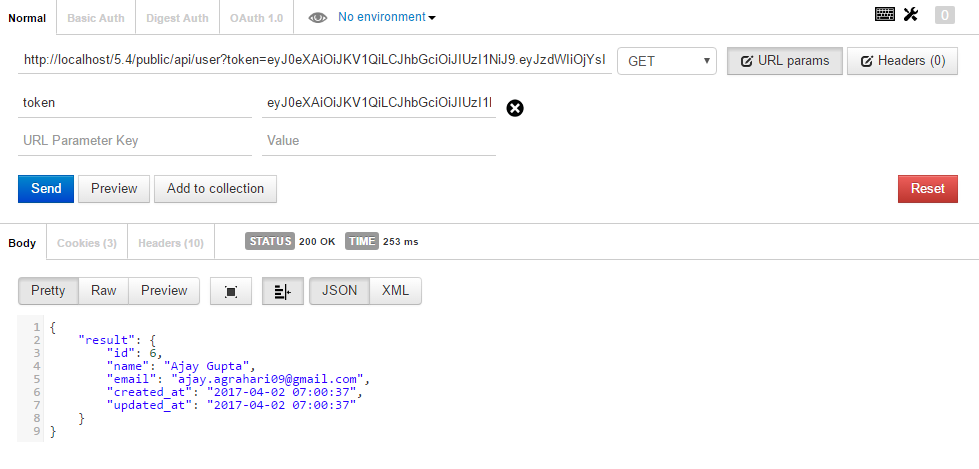
4 : If you pass the invalid token then you will get following response :
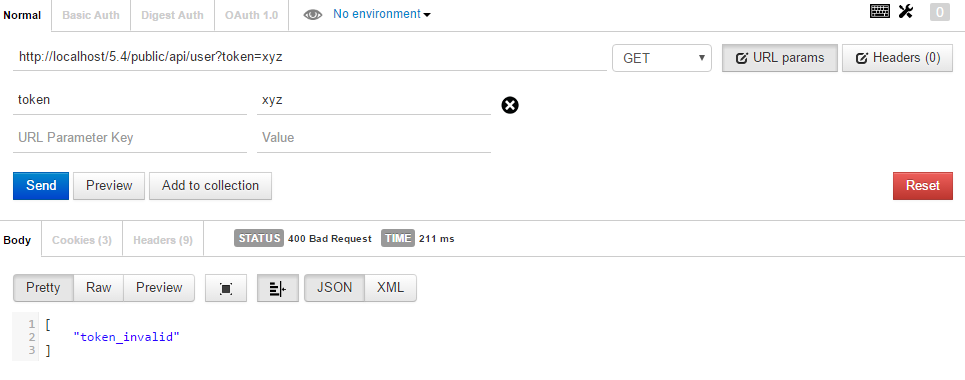
Click here to know the use of JWT in Node.js
Generate JWT token after login and verify with Node.js API