Generate JWT token after login and verify with Node.js API
In my last tutorial, I explained about how to login and register the user in the Node.js without using any security token.
But now I will tell you how to create a token using JWT library and authenticate APIs using the generated token in Node.js application.
Authentication, is what? It is just the way to keep unauthorized users out from accessing your secure APIs to interact with the database. Authentication is a main concern of the secure application.
Token based authentication is called stateless and session less because whenever we authenticate any user then we do not save any user details on the server, We simply generate a token that is signed by a private key and send it to the user to authenticate all secure api with generated token.
In this post, The flow of authenticate a token is as follows :
- Verify username and password from the database.
- Generate a JWT token if user credentials match with database record and send it to the client.
- The client saves token and sends it with all secure APIs.
- Use middleware to verifies the token and process the request.
You can send token in any way but this is good practice to send token in an HTTP header.
Getting StartedBefore going with the steps, Make sure you have installed node and npm
.
Let's have a look at our directory structure for Node application.
Directory Structure
- ├── controllers
- │ └── authenticate-controller.js
- ├── node_modules
- ├── config.js
- ├── index.js
- └── package.json
package.json
is a beginning file for node application where you can see the list installed packages in your node application.
{ "name": "jwt", "version": "1.0.0", "description": "jwt authentication", "main": "index.js", "dependencies": { "body-parser": "^1.17.1", "express": "^4.14.1", "jsonwebtoken": "^7.3.0", "mysql": "^2.13.0" }, "devDependencies": {}, "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "", "license": "ISC" }
express
module is the very popular Node framework.
body-parser
module is used to get data from POST request.
jsonwebtoken
module is used to generate and authenticate our JSON Web Tokens.
mysql
module is how you work with database.
In this step, we will create a connection object that will used with every database query.
config.js
- var mysql = require('mysql');
- var connection = mysql.createConnection({
- host : 'localhost',
- user : 'root',
- password : '',
- database : 'test'
- });
- connection.connect(function(err){
- if(!err) {
- console.log("Database is connected");
- } else {
- console.log("Error while connecting with database");
- }
- });
- module.exports = connection;
In this step, we will include the packages we installed.
- var express=require("express");
- var bodyParser=require('body-parser');
- var jwt= require("jsonwebtoken");
- var app = express();
- var router=express.Router();
- var authenticateController=require('./controllers/authenticate-controller');
- process.env.SECRET_KEY="thisismysecretkey";
- app.use(bodyParser.urlencoded({extended:true}));
- app.use(bodyParser.json());
- app.post('/api/authenticate',authenticateController.authenticate);
- app.use('/secure-api',router);
- // validation middleware
- router.use(function(req,res,next){
- var token=req.body.token || req.headers['token'];
- if(token){
- jwt.verify(token,process.env.SECRET_KEY,function(err,ress){
- if(err){
- res.status(500).send('Token Invalid');
- }else{
- next();
- }
- })
- }else{
- res.send('Please send a token')
- }
- })
- router.get('/home',function(req,res){
- res.send('Token Verified')
- })
- app.listen(8012);
In above code, i use middleware to protect last API prefix with 'secure-api' (localhost:8012/secure-api/home).
Create Authenticate Controller to generate tokenIn above code, I have defined a route to handle post request from the url localhost:8012/api/authenticate
and respectively I have called authenticate method which is defined into authenticate controller.
So let's create a authenticate-controller.js, Where we validate user details and then generate a token.
controllers/authenticate-controller.js
- var jwt=require('jsonwebtoken');
- var connection = require('./../config');
- module.exports.authenticate=function(req,res){
- var email=req.body.email;
- var password=req.body.password;
- connection.query('SELECT * FROM users WHERE email = ?',[email], function (error, results, fields) {
- if (error) {
- res.json({
- status:false,
- message:'there are some error with query'
- })
- }else{
- if(results.length >0){
- if(password==results[0].password){
- var token=jwt.sign(results[0],process.env.SECRET_KEY,{
- expiresIn:5000
- });
- res.json({
- status:true,
- token:token
- })
- }else{
- res.json({
- status:false,
- message:"Email and password does not match"
- });
- }
- }
- else{
- res.json({
- status:false,
- message:"Email does not exits"
- });
- }
- }
- });
- }
Now start the server by running following command :
node index.jsTest Our API to Authenticate User and Create a Token with Postman
First, i will hit api localhost:8012/secure-api/home
without sending any token to test our middleware.
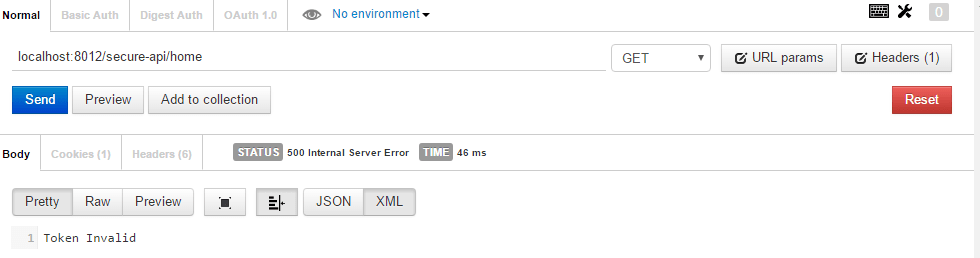
Now let's send the token with request.