Simple and Easy Laravel 5.2 Login and Register using the auth scaffold
Here i am going to use Laravel auth scaffolding to login and register, its very easy to integrate login and register system in Laravel.
Step 1: Install Laravel 5.2If Laravel is not installed in your system then first install with following command and get fresh Laravel project.
composer create-project --prefer-dist laravel/laravel blog "5.2.*"
When you run above command then it create application with name blog in your system.From Laravel 5, you have to install laravelcollective/html for Form class.To know the installation process of laravelcollective/html, kindly go through this url HTML/FORM not found in Laravel 5?.
Step 2: Create users table and modelFollow the simple step to create users table in your database.First create migration for users table using Laravel 5 php artisan command,so first run this command -
php artisan make:migration create_users_table
After this command, you will see a migration file in following path database/migrations and you have to simply put following code in migration file to create users table.
- use Illuminate\Database\Schema\Blueprint;
- use Illuminate\Database\Migrations\Migration;
- class CreateUsersTable extends Migration
- {
- /**
- * Run the migrations.
- *
- * @return void
- */
- public function up()
- {
- Schema::create('users', function (Blueprint $table) {
- $table->increments('id');
- $table->string('name');
- $table->string('email')->unique();
- $table->string('password');
- $table->rememberToken();
- $table->timestamps();
- });
- }
- /**
- * Reverse the migrations.
- *
- * @return void
- */
- public function down()
- {
- Schema::drop('users');
- }
- }
Save this migration file and run following command
php artisan migrate
If you receive a "class not found" error when running migrations, try running the composer dump-autoload
command and re-issuing the migrate command.
Now you have successfully created `users` table.You won't have to create user model, it is created by default in following path app/User.php when you install Laravel.
- namespace App;
- use Illuminate\Foundation\Auth\User as Authenticatable;
- class User extends Authenticatable
- {
- /**
- * The attributes that are mass assignable.
- *
- * @var array
- */
- protected $fillable = [
- 'name', 'email', 'password',
- ];
- /**
- * The attributes that should be hidden for arrays.
- *
- * @var array
- */
- protected $hidden = [
- 'password', 'remember_token',
- ];
- }
Laravel provide a short cut to create auth section
php artisan make:auth
After successfully run command, Laravel create complete auth section which you will see in your command promt -
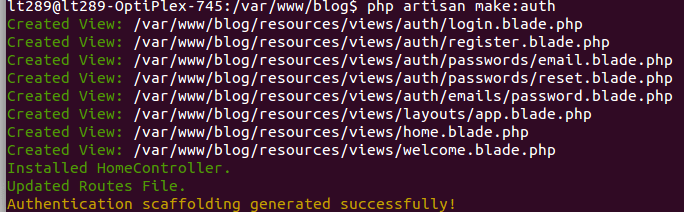
List of files and folder which is generated by above command :
It generate a master layout which is core of scaffold resources/views/layouts/app.blade.php
List of views that extend it :
- welcome.blade.php - the welcome page
- home.blade.php - the landing page for logged-in users
- auth/login.blade.php - the login page
- auth/register.blade.php - the register or signup page
- auth/passwords/email.blade.php - the password reset confirmation page
- auth/passwords/reset.blade.php - the password reset prompt page
- auth/emails/password.blade.php - the password reset email
Changes in routes.php :
- Route::auth();
- Route::get('/home', 'HomeController@index');
The Route::auth()
is a set of common authentication, registration and password reset routes.
- // Authentication Routes...
- $this->get('login', 'Auth\AuthController@showLoginForm');
- $this->post('login', 'Auth\AuthController@login');
- $this->get('logout', 'Auth\AuthController@logout');
- // Registration Routes...
- $this->get('register', 'Auth\AuthController@showRegistrationForm');
- $this->post('register', 'Auth\AuthController@register');
- // Password Reset Routes...
- $this->get('password/reset/{token?}', 'Auth\PasswordController@showResetForm');
- $this->post('password/email', 'Auth\PasswordController@sendResetLinkEmail');
- $this->post('password/reset', 'Auth\PasswordController@reset');
Benefits of using scaffolding in Laravel is you don't have to create routes, controllers and views for login and register.It helps you to save 30 to 60 minutes of typing on every app that needs it.
Now let's have a look at what we get in browser.