Understanding AngularJS Controllers and scope inheritance with example
Controllers are used to basically control the data in any MVC pattern application, In same way with AngularJS Controller control the flow of data in the application.
Controllers in AngularJS is attached to the DOM by using ng-controller
directive.
Never use controllers to manipulate DOM because as per MVC standard controllers should handle only business logic.
You can follow the approach of AngularJS's databinding feature in your application to synchronize data automatically between model and view components.
A controller in AngularJS is defined by a JavaScript constructor function and when controller is attached with DOM by using ng-controller
directive then it will instantiate a new object of controller and a new child scope($scope) will be created.
I here let you know about scopes What is scope is nothing only hold the Model data to be passed into view. In simple word it is an object that uses two way data binding to bind model data with view.
Let's go with simple example to understand about controllers and their work :
- <!DOCTYPE html>
- <html ng-app="myApp">
- <head>
- <title>Understanding AngularJS Controllers</title>
- <script type="text/javascript"
- src="http://ajax.googleapis.com/ajax/libs/angularjs/1.0.7/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="myController">
- Welcome to {{ myName }}
- </div>
- <script type="text/javascript">
- angular.module("myApp", []).controller("myController", function($scope) {
- $scope.myName='ExpertPHP.in';
- });
- </script>
- </body>
- </html>
You can see in above example, application is defined in AngularJS by using ng-app="myApp".
Using ng-controller
you can define controllers in AngularJS and myController is JavaScript function and it invoke a $scope object.
Using $scope object we bind the string 'ExpertPHP.in' with myName and this property is now bound to the template.
Another Example: Adding Behavior to a Scope Object
- <!DOCTYPE html>
- <html ng-app="myApp">
- <head>
- <title>Understanding AngularJS Controllers</title>
- <script type="text/javascript"
- src="http://ajax.googleapis.com/ajax/libs/angularjs/1.0.7/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="SquareController">
- Square of <input ng-model="num"> is equals {{ square(num) }}
- </div>
- <script type="text/javascript">
- var app = angular.module('myApp',[]);
- app.controller('SquareController', ['$scope', function($scope) {
- $scope.square = function(value) { return value * value; };
- }]);
- </script>
- </body>
- </html>
ng-model
directive is used to bind the value of input field to a variable.
And call square method in an Angular expression in the template/view and add behavior to the scope $scope object by adding this method.
Call Controller Method on ng-click
- <!DOCTYPE html>
- <html ng-app="myApp">
- <head>
- <title>Understanding AngularJS Controllers</title>
- <script type="text/javascript"
- src="http://ajax.googleapis.com/ajax/libs/angularjs/1.0.7/angular.min.js"></script>
- </head>
- <body>
- <div ng-controller="SquareController">
- <input ng-model="num"> Square of {{ num }} is equals {{total}}
- <button ng-click="square()">Square</button>
- </div>
- <script type="text/javascript">
- var app = angular.module('myApp',[]);
- app.controller('SquareController', ['$scope', function($scope) {
- $scope.num = 5;
- $scope.square = function() { $scope.total= $scope.num * $scope.num; };
- }]);
- </script>
- </body>
- </html>
ng-click
directive allow you to add custom behavior when any element is clicked and in above example i use ng-click to binds the click event on the button to get square value entered in input box so whenever button is clicked, the square method on $scope will be called.
- <!DOCTYPE html>
- <html ng-app="myApp">
- <head>
- <title>Understanding AngularJS Controllers</title>
- <script type="text/javascript"
- src="http://ajax.googleapis.com/ajax/libs/angularjs/1.0.7/angular.min.js"></script>
- </head>
- <body>
- <div class="aboutme">
- <div ng-controller="FirstController">
- <p>Hi, I am {{name}} and my age {{age}}</p>
- <div ng-controller="SecondController">
- <p>Hi, I am {{name}} and my age {{age}}</p>
- <div ng-controller="ThirdController">
- <p>Hi, I am {{name}} and my age {{age}}</p>
- </div>
- </div>
- </div>
- </div>
- <script type="text/javascript">
- var app = angular.module('myApp',[]);
- app.controller('FirstController', ['$scope', function($scope) {
- $scope.name = 'Ajay';
- $scope.age = '25';
- }]);
- app.controller('SecondController', ['$scope', function($scope) {
- $scope.name = 'Vikash Kumar';
- }]);
- app.controller('ThirdController', ['$scope', function($scope) {
- $scope.name = 'Vikash Verma';
- $scope.age = '32';
- }]);
- </script>
- </body>
- </html>
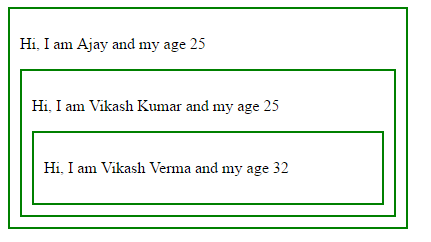
You can see in above example how we nested three ng-controller in template:
- The FirstController that contains name and age both properties.
- SecondController that contains name only and inherit age property from the previous.
- ThirdController that overrides both the name property defined in SecondControler and the age property defined in FirstController.