In this Codeigniter 3 Tutorial, I will let you know about RESTful web services.
RESTful we services are a medium to exchange data between applications or system on the internet.
RESTful web services are used for creating APIs because they are lightweight and loosely coupled web services. With the help of APIs, you can create a bridge between backend system and modern mobile application.
RESTful web service uses HTTP methods like get, post, put and delete.
Today we'll know how we can create a RESTful API in Codeigniter in order to exchange data between different applications.
As we know, Codeigniter is a PHP based web application framework and for the beginners it's very easy and simple to learn.
In this example, I will create a rest apis for "users" module in Codeigniter 3 application.
Step 1: Create products TableIn order to create restful web services, we need to create a table first in MySQL database.
CREATE TABLE `products` ( `id` int(10) unsigned NOT NULL AUTO_INCREMENT, `name` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `price` double NOT NULL, `created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP, `updated_at` datetime DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=17 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ciStep 2: Configure REST Controller Library
In this step, we will configure the REST Controller Library in our Codeigniter application.
Below are the few steps to setup REST Controller :
- Download the Rest config file and place that file in the
application/config/
directory. - Download the REST_Controller.php and Format.php file and place that files in the
application/libraries/
directory. - Download the Language file and place that file in the
application/language/english/
directory.
Update the application/config/config.php
from $config['subclass_prefix'] = 'MY_'; to : $config['subclass_prefix'] = 'REST_';
In this step, I will create a api folder in the controllers diectory and inside the api folder create a file "Product.php" and put the below code into that file.
application/controllers/api/Product.php<?php require APPPATH . '/libraries/REST_Controller.php'; use Restserver\Libraries\REST_Controller; class Product extends REST_Controller { /** * Get All Data from this method. * * @return Response */ public function __construct() { parent::__construct(); $this->load->database(); } /** * Get All Data from this method. * * @return Response */ public function index_get($id = 0) { if(!empty($id)){ $data = $this->db->get_where("products", ['id' => $id])->row_array(); }else{ $data = $this->db->get("products")->result(); } $this->response($data, REST_Controller::HTTP_OK); } /** * Get All Data from this method. * * @return Response */ public function index_post() { $input = $this->input->post(); $this->db->insert('products',$input); $this->response(['Product created successfully.'], REST_Controller::HTTP_OK); } /** * Get All Data from this method. * * @return Response */ public function index_put($id) { $input = $this->put(); $this->db->update('products', $input, array('id'=>$id)); $this->response(['Product updated successfully.'], REST_Controller::HTTP_OK); } /** * Get All Data from this method. * * @return Response */ public function index_delete($id) { $this->db->delete('products', array('id'=>$id)); $this->response(['Product deleted successfully.'], REST_Controller::HTTP_OK); } }
You can test the apis through Postman Extension :
Product List API :
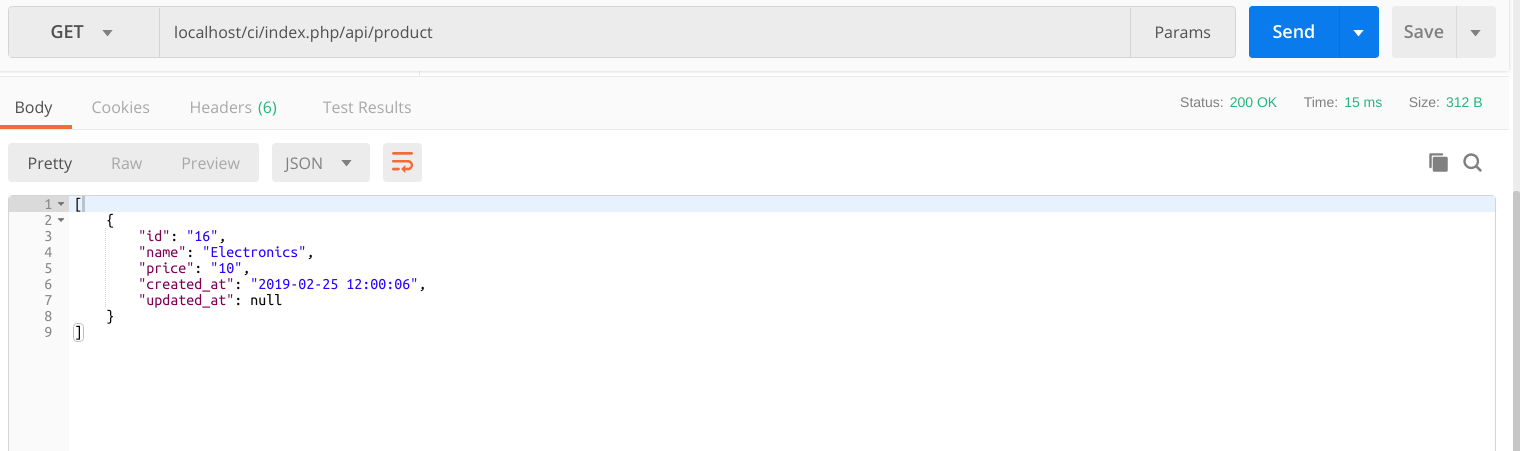
Add New Product API :
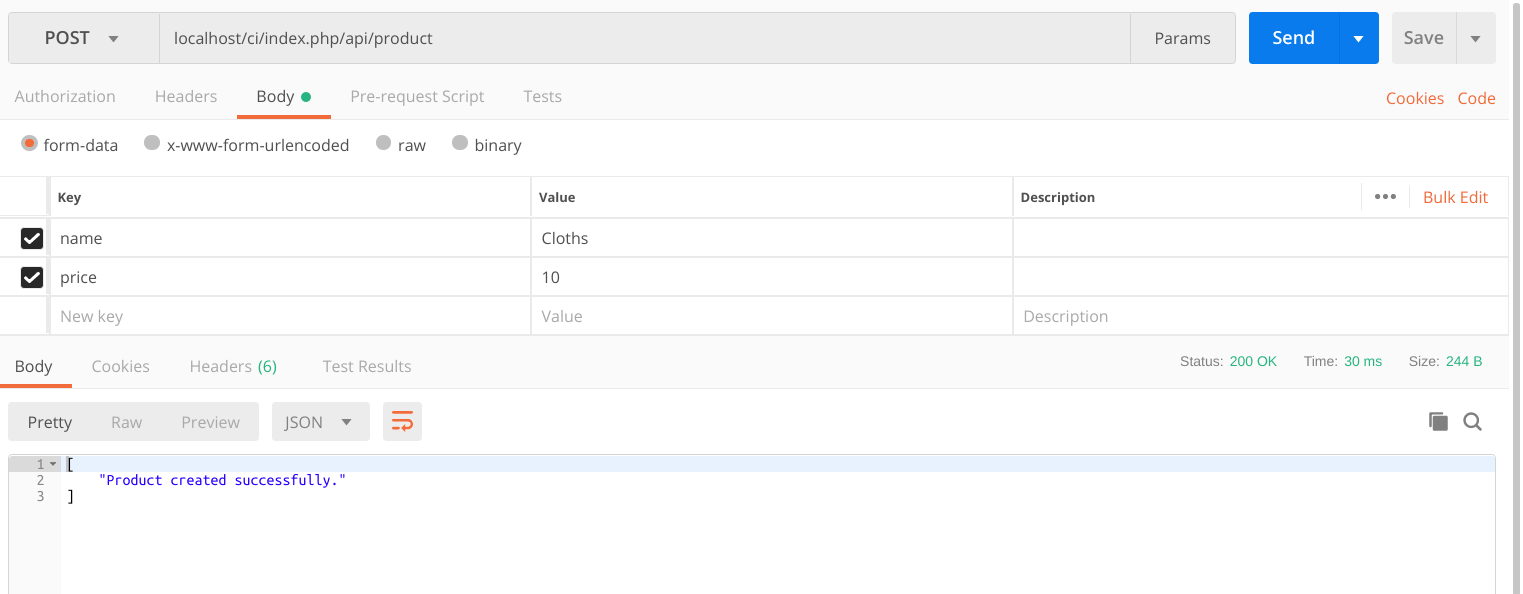
View Product Details API :
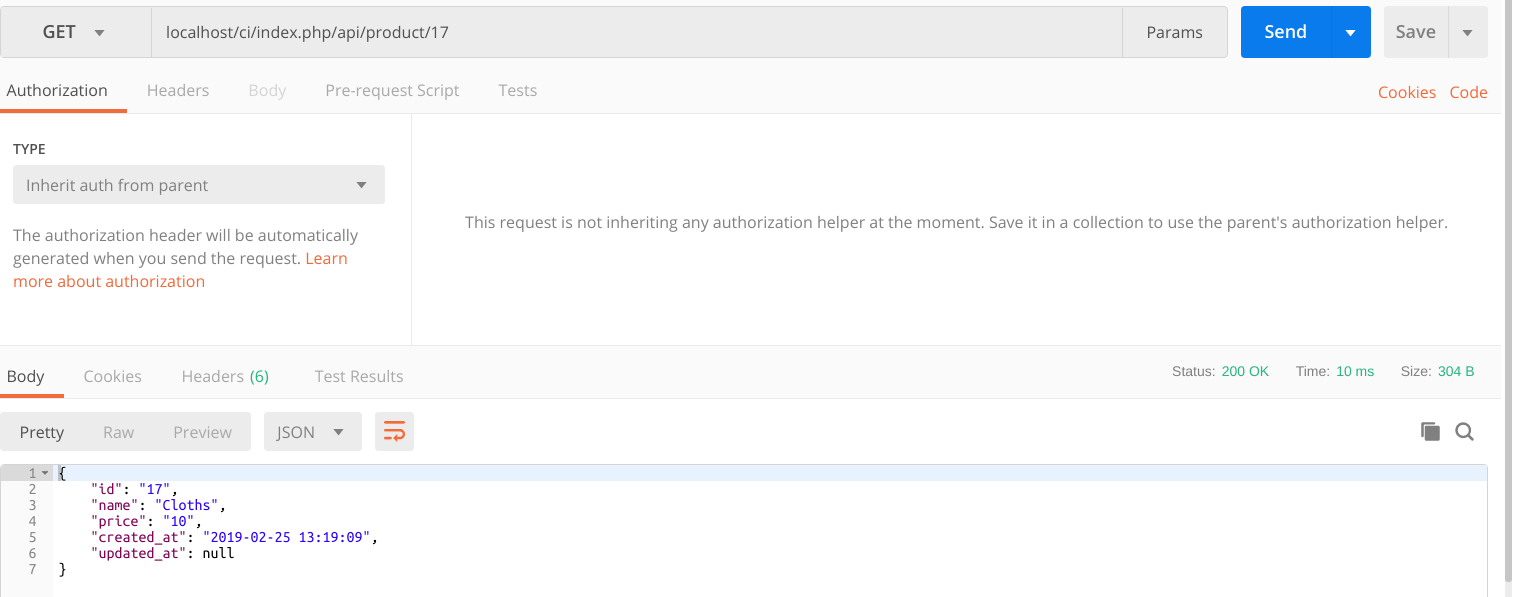
Update Product Details API :
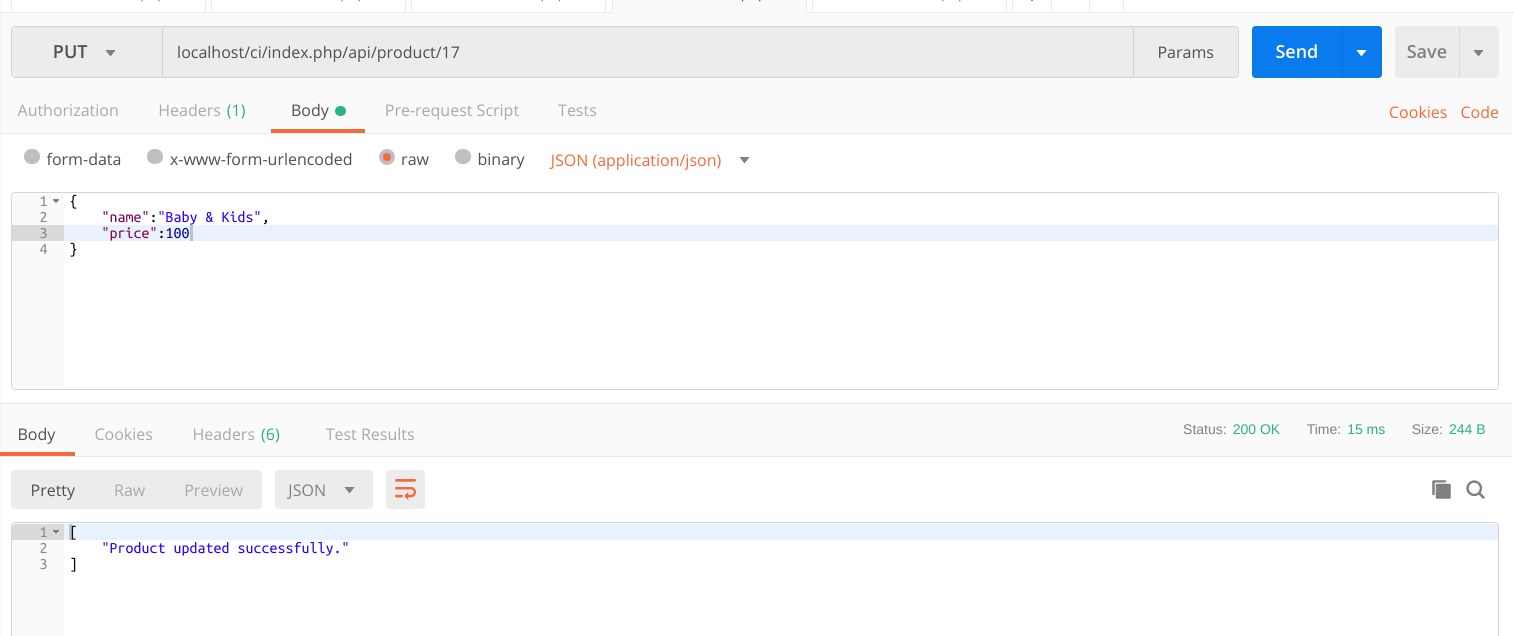
Delete Product Data API :
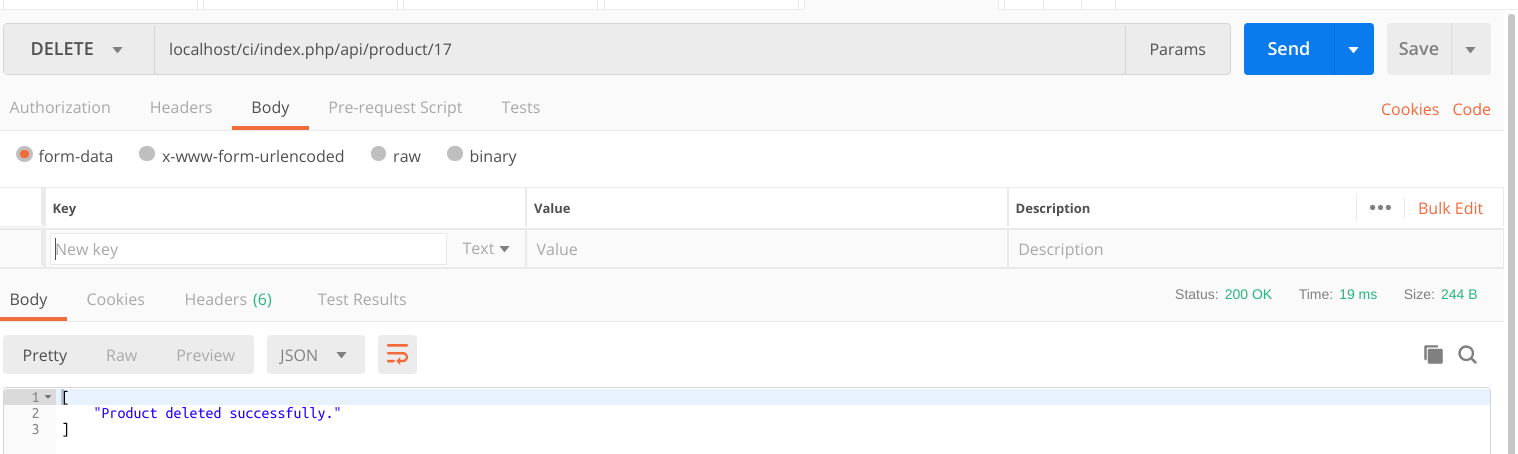